Mobile - React Native
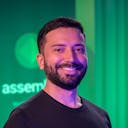
React Native
React Native is a framework for building mobile apps using React. It allows developers to create native apps for Android and iOS using only JavaScript and React.
React Native has a new architecture called Fabric, which was released in 2019. Fabric completely refactors React Native's core for better modularity, stability and performance.
The main Fabric changes include:
- New Thread Model - More efficiently manages UI, memory handling and serialization threads.
- Modular Architecture - The core was split into independent modules, allowing incremental updates.
- Backwards Compatibility - Fabric maintains compatibility with older apps.
- Enhanced Performance - Memory management, start-up time and CPU usage were optimized.
- Updated Build System - Uses C++ bindings instead of Java, resulting in smaller and faster apps.
- Fabric is being gradually adopted and will become React Native's standard architecture over the next few years.
EXPO
Expo is a tool and framework built on top of React Native that allows you to develop native apps for iOS, Android and web more quickly and easily.
The main Expo features and advantages are:
- No native configuration required - Expo abstracts away the complexity of building and deploying native apps to app stores.
- Ready libraries - Easy access to various native APIs and services like camera, location, push notifications, OpenGL etc.
- Hot Reloading - Instantly updates the app under development with code changes.
- OTA (over-the-air) Builds - Enables distributing test versions of the app directly from Expo without going through app stores.
- Tool integration - Integration with Expo CLI, servers, popular dependencies and libraries.
- Active community - Being open source, it has a large community behind it.
Expo Go
- Uses Expo SDK and libraries. No custom native code access.
- Builds and serves OTA updates through Expo servers.
- Limited configuration - uses app.json and app.config.js.
- Great for quick prototyping and iteration.
- Some limitations on advanced native features.
Expo Development Build
- Uses Expo SDK but allows custom native code.
- Requires prebuild to generate native projects.
- Full access to native APIs and config.
- Can use most libraries with proper configuration.
- Builds through EAS Build, not Expo servers.
- More configuration required but full control.
Bare Workflow
- Completely custom native project, no Expo SDK.
- Manually configure native Xcode and Android Studio projects.
- Full control over all code, dependencies, config.
- No limits on native APIs, libraries, or tooling.
- No Expo services benefits like OTA updates.
- Most configuration and build/deploy work required.
In summary:
- Expo Go is the easiest but most limited.
- Development builds allow native access with Expo.
- Bare workflow is a pure React Native project without Expo.
React Native Limitations
-
Single threaded rendering can cause sluggishness, especially during animations or when running multiple processes. Extensions like bridges to native components can allow some multi-threading.
-
Slow browser transitions occur when trying to render new screens during existing animations. Using InteractionManager API methods like runAfterInteractions() can delay operations until animations finish.
-
Memory leaks occur due to issues like improper handling of closures capturing variables. Setting references to null once finished can allow proper garbage collection.
-
Overall, React Native blends web and native development well, and though limitations exist, developers can avoid or optimize most performance problems with careful coding and testing on real devices. Thoughtfully architecting complex apps and using methods like InteractionManager can create smooth UIs.
Simulator vs Emulator
iOS Simulator:
- Simulates the iOS environment and Apple devices (iPhone, iPad) on a Mac.
- It is faster and more fluid given its native integration with the OS.
- Allows testing native iOS features like CoreLocation, iCloud etc.
- Doesn't allow testing some hardware like camera, GPS etc.
Android Emulator:
- Emulates Android devices by running a virtual machine on any OS.
- It is slower due to virtualization, consumes more resources.
- Allows testing hardware features like camera, GPS, sensors etc.
- Can have incompatibilities with some newer Android features.
- Allows configuring a wide variety of devices, OS versions and features.
Notifications
React Native has a Notifications API that provides access to the native notification system on each platform. This allows creating and scheduling notifications locally and remotely.
- On Android, you can use Firebase Cloud Messaging (FCM) to send remote notifications to the device. FCM has good integration with React Native.
- On iOS, you can use Apple Push Notification Service (APNS) similarly for remote notifications. Push notifications have more features on iOS.
- For both platforms, you need to request user permission to send notifications. This is handled by React Native's Notifications API.
- Notification options like icons, sounds and vibration can be customized on both platforms. On iOS there are more advanced options.
- The content, appearance and behavior of notifications when received can be customized on each OS.
- Scheduling local time or location based notifications is possible on both through the Notifications API.
Performance
To evaluate our app's performance we have some tools to assist us:
- The developer menu provides debug options like Fast Refresh, LogBox and Performance Monitor. Enabling them helps detect issues.
Understanding FPS (Frames per Second): Maintaining a consistent FPS rate of 60 frames per second creates a smooth user experience. FPS drops cause lag and sluggishness.
Common reasons for performance issues (Low FPS): Development mode, console.log, large ListView, unnecessary re-rendering and complex animations can reduce FPS.
4 React Native Threads Main Thread, JavaScript Thread, Native Modules Thread and Render Thread (Android 5+) play distinct roles. Understanding which thread causes bottlenecks helps.
Profiling measures runtime time and memory complexity. This helps track and provide solutions to keep the app healthy.
- Profiling on iOS: Xcode, Instruments and Flipper + Hermes Debugger RN are profiling tools for iOS. They help detect performance bottlenecks.
- Profiling on Android: Android Profiler, System Tracing and Flipper plugin perform a similar function for Android. Identifying high cost operations and optimizing them improves performance.
FlatList https://blog.stackademic.com/react-native-masters-4-optimizing-flatlist-performance-5204ab6751cf
Security
You can check my article about security in the mobile world here.
DB - Mobile databases
- Built-in key-value storage for React Native.
- Allows saving simple data on the device asynchronously.
- Good for simple data that doesn't require complex queries.
- Mobile database optimized for performance.
- Replaces SQLite with more Javascript friendly types and queries.
- Offers sync between devices and support for modern features.
- Lightweight relational database built into Android and iOS platforms.
- Offers more features than Async Storage like complex types and SQL queries.
- Requires Javascript wrappers like SQLite or Android SQLite.
- Reactive mobile database focused on performance and dev experience.
- Uses SQLite or Async Storage as the storage engine.
- Easy to use reactive APIs.
- Lightweight open source state management library for React Native.
- Integrated data persistence powered by Async Storage or SQLite.
- Declarative data fetching and caching system.
- Integrates nicely with React Native ecosystem.
- Focused on performance and small build size.
- Good for apps where speed and size are critical.
Deployment
Fastlane
- Fastlane is an open source command line tool for automating deployment and distribution tasks for iOS and Android apps.
- It has built-in support for React Native and can handle code signing, profile provisioning, app building, uploads to App Store and Play Store among other tasks.
- Fastlane uses special files called Fastfile that define automation scripts with the desired actions. This allows creating reproducible deploy workflows.
- Some Fastlane benefits are easy setup, integration with CI services, and huge time savings by automating a complex manual process.
Bitrise
- Bitrise is a mobile-focused Continuous Integration and Delivery platform online.
- It has dedicated support for React Native with various tools and guides to set up automated deploy processes.
- Bitrise runs in the cloud and manages building, testing and deploying your apps from a Git repository with the source code.
- Some advantages are deep integration with app storage and distribution services, visual build history monitoring, and streamlined collaboration for teams.
Staged Rollout
Practice of releasing new features or app updates gradually to a subset of users before making it available to everyone.
Allows testing updates with a smaller group first before a full rollout. Helps identify and fix issues early on.
iOS
Supports staged rollouts via TestFlight. Developers can submit a beta build and add testers.
The build is available to testers for a set timeframe or until promoted to a full App Store release.
New versions can be made available incrementally by user percentage or specific country.
Android
Supports staged rollouts via Google Play Console and closed alpha/beta tester groups.
New versions can be made available incrementally by user percentage or specific country.
Monitoring
Using Datadog RUM - Real User Monitoring.
Embedded content: https://docs.google.com/presentation/d/13i6DSTER34ukxye61-4mYfPS6QxSBt1TELs0WZErUiA/edit?usp=sharing