GraphQL
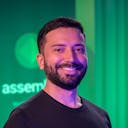
GraphQL: A Journey from Pain Points to Modern API Development
The Origin Story
Back in 2012, Facebook was facing a significant challenge with their mobile applications. The REST APIs they had built were becoming increasingly inefficient at serving data to their complex, rapidly evolving mobile interfaces. Engineers found themselves constantly modifying backend endpoints to accommodate new feature requirements, leading to numerous API versions and a frustrating development experience.
Enter Lee Byron, Nick Schrock, and Dan Schafer – the minds behind GraphQL. They set out to create a query language that would solve Facebook's mobile data fetching woes. By 2015, Facebook open-sourced GraphQL, and it quickly gained traction in the developer community.
The Core Concept: Why GraphQL?
GraphQL addresses several fundamental pain points in API development:
1. Over-fetching and Under-fetching
With REST, you often get back more data than you need (over-fetching) or need to make multiple requests to different endpoints to get all the data you want (under-fetching). GraphQL solves this with a single query that precisely specifies the data you need:
query {
user(id: "123") {
name
email
posts {
title
publishedDate
}
}
}
2. Multiple Round Trips
Instead of making several REST calls to different endpoints, GraphQL allows you to fetch related data in a single request:
query {
movie(id: "456") {
title
director {
name
otherMovies {
title
year
}
}
cast {
name
character
}
}
}
3. API Evolution
Unlike REST APIs where adding new fields requires new versions or endpoints, GraphQL APIs can evolve without breaking existing queries. Clients only request the fields they need, so new fields can be added without affecting existing clients.
Modern GraphQL Tooling Ecosystem
The GraphQL ecosystem has matured significantly since its inception. Here are some essential tools:
Development Tools
- Apollo Server: The most popular GraphQL server implementation for Node.js
- GraphQL Yoga: A fully-featured GraphQL server with focus on easy setup
- Prisma: Modern database toolkit that works seamlessly with GraphQL
- GraphQL Code Generator: Automating code generation for GraphQL operations
Client Libraries
- Apollo Client: Comprehensive state management library for GraphQL
- urql: Lightweight and extensible GraphQL client
- Relay: Facebook's own GraphQL client with strong focus on performance
Developer Experience
- GraphQL Playground: Interactive IDE for exploring GraphQL APIs
- GraphiQL: The original in-browser IDE for GraphQL
- Apollo Studio: Professional platform for GraphQL API management
Quick Implementation Example
Here's a simple GraphQL server implementation using Apollo Server:
const { ApolloServer, gql } = require('apollo-server');
// Schema definition
const typeDefs = gql`
type Book {
id: ID!
title: String!
author: Author!
}
type Author {
id: ID!
name: String!
books: [Book!]!
}
type Query {
books: [Book!]!
author(id: ID!): Author
}
`;
// Resolver implementations
const resolvers = {
Query: {
books: () => books,
author: (_, { id }) => authors.find(author => author.id === id)
},
Book: {
author: (book) => authors.find(author => author.id === book.authorId)
},
Author: {
books: (author) => books.filter(book => book.authorId === author.id)
}
};
// Server initialization
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`🚀 Server ready at ${url}`);
});
Looking Forward
GraphQL continues to evolve with exciting developments:
-
Federation: Apollo Federation enables teams to split GraphQL services into smaller, more manageable parts while still presenting a unified API to clients.
-
Subscriptions and Live Queries: Real-time capabilities are becoming more sophisticated, enabling better live data features.
-
Edge Computing: GraphQL is adapting to edge computing scenarios, with tools like GraphQL Mesh and GraphQL Helix enabling new deployment patterns.
Conclusion
GraphQL has transformed from a solution to Facebook's mobile data fetching problems into a robust ecosystem for building flexible, efficient APIs. While it's not a silver bullet for all API needs, its developer experience, tooling, and community make it an excellent choice for modern applications dealing with complex data requirements.
The initial learning curve may be steep, but the benefits of precise data fetching, strong typing, and excellent tooling make it a worthwhile investment for teams building data-intensive applications. As the ecosystem continues to mature, we can expect even more innovations in how we build and consume APIs using GraphQL.